みなさんこんにちは、パパゴンです。
今回の記事ではゲーム作成の記事になります。
みなさん副業でゲーム作成してみたいと思ったことありませんか?
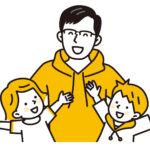
実は僕自信も思ったことはありますけど、プログラムとか難しい…ってなって諦めたことがわかるんです。
でも今はAIの時代!
そんなプログラムは全部AIに任せたら無料+簡単にゲームが作れました。
僕自身もAIでゲーム作るのは初めてなので、簡単なテトリスから作ってみました。
今回の記事では、AIを使ってテトリスの作成方法を教えます。
この記事を見ながら作れるようにしますので、読者の方々も一緒に作ってみませんか?
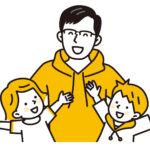
序盤は少し難しい話になりますが、そんなんいらん!作り方だけを知りたいって方は目次の作り方の方にお飛び下さい。
今回の記事でわかること!!
・ゲーム作成ツール
・AIツールの紹介
・プログラミング言語について
・ゲームの作り方
・ゲームの公開先
ゲーム作成時に知っておいた方がいいこと(初心者必見)
そもそも、初心者でもゲームができるの?
プログラミング言語も何もわからないよ?と思われている方でも、今回の記事を真似するだけでゲームが作れます!
今回のやり方では、無料でゲーム制作ができますので、お試しで一度遊び感覚でゲームが作れます。
ゲーム開発ツール
基本的に今から紹介するゲーム制作ツールは無料のものとなります。
- Unity (無料&有料)
- Unreal Engine (無料)
- Cocos2d-x (無料)
- replit (無料&有料)
ちなみに、今回使用したのは 【 replit 】です。
後ほど、使い方の説明もします!!
Unity
-1024x392.png)
Unity Student 【 無料 】
Unity Personal 【 無料 】
Unity Pro 【 29700円 】
Unreal Engine
-1024x465.png)
基本プレイ 【 無料 】
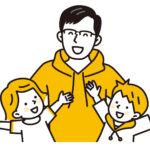
無料で使えますが、収益化は100万米ドル(1億4397万円)の売り上げが出るまでは、製作者にお金は入ってきません。
Cocos2d-x
-1024x468.png)
基本プレイ【 無料 】
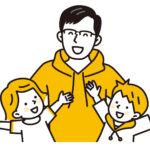
商品利用も無料で可能です
replit
-1024x470.png)
- Starter 【 無料 】
- Replit Core 【 20ドル=2900円ほど 】になります。
- Teams 【 35ドル=5600円 】になります。
コードを教えてくれるAIツール
- chat GPT(無料)
- gemini(無料)
- GitHub Copilot(有料)
- DeepCode(無料&有料)
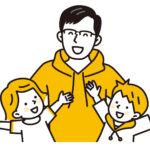
今回私が使用したのは、【 chat GPT 】ですが、他にも色々あるので使ってみてください。
最初は無料でも通用するとは思いますが、より高度なコードが必須になってくると、有料の方がいいかもしれないですね!
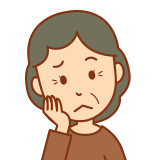
コードってなに?なんにもわかんないよ~
AIに出してもらう、コードとは
今回AIに出してもらう、HTML、CSS、JavaScriptは、Webサイトやアプリケーションを作成するための3つの主要な技術です。
それぞれをなるべくわかりやすく簡単に説明します。
HTML (HyperText Markup Language)
Webページの構造と内容を定義します。
テキスト、画像、リンクなどの要素を配置します。
「タグ」を使って要素を記述します。
CSS (Cascading Style Sheets)
HTMLの見た目を整えます。
色、サイズ、レイアウトなどのデザインを指定します。
HTMLと分離して、スタイルを一元管理できます。
JavaScript
Webページに動きと対話性を追加します。
ユーザーの操作に応じて内容を変更できます。
複雑な機能やアニメーションを実現します。
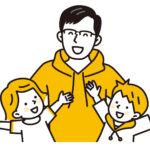
一応説明しましたが、こんな知識まったくいりません!
では早速、作ってみましょう!!
作り方
作り方はたったの3ステップ!!
- AIに指示を出し
- 出されたコードを指定の場所に貼り付け
- 修正
この三つをするだけでゲームが完成します。
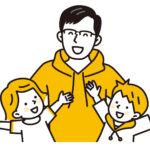
※今回使用するツールは【 chat GPT 】【 replit 】
AIに指示
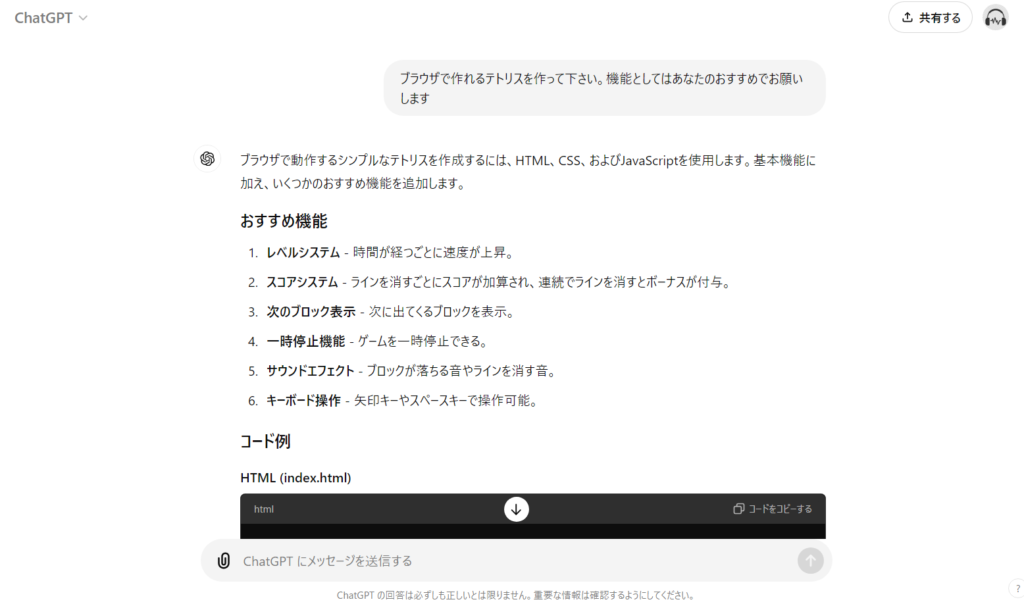
こんな感じで、AIに命令します。
そうすると、プログラミングコードをだしてきます。
命令文
ブラウザで作れるテトリスを作ってください。機能としてはあなたのおすすめでおねがいします。
⇧このように指示してください。
そうすると、HTML,CSS,JavaScriptをだしてくれます。
一回で、完璧なコードを出してくれないこともあるので、その都度AIに指示してください。
コードを貼る
次に一度コードをコピーして、【 replit 】に貼り付けましょう。
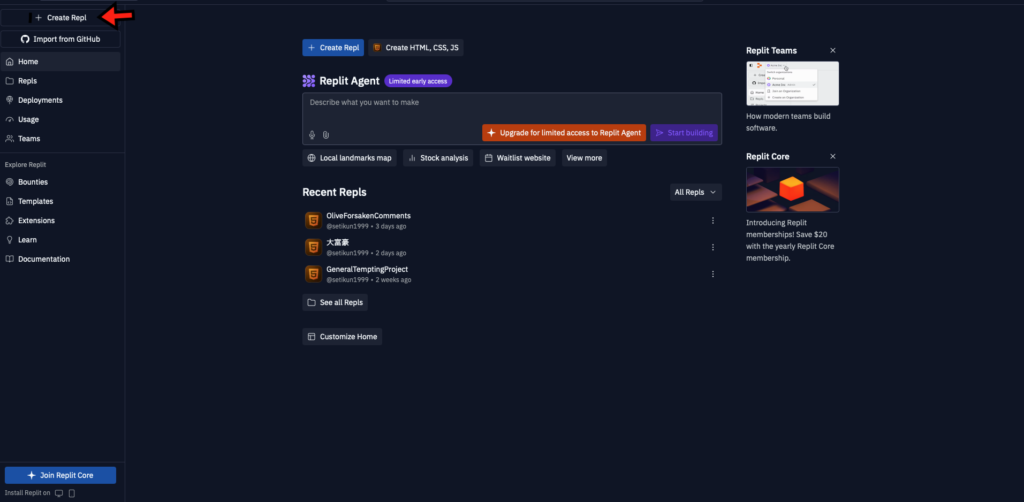
そして、左上の+Create Real押しゲームを作成しましょう。
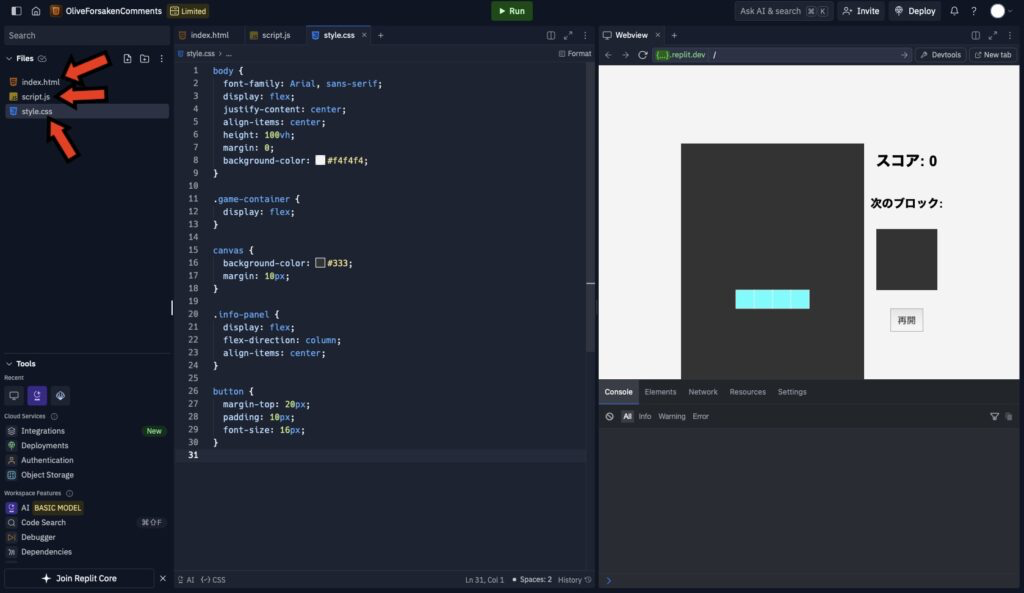
そして次に、【 index, html 】【 script,js 】【 style, css 】にそれぞれのコードを貼り付けましょう!
HTMLコード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>テトリス</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="game-container">
<canvas id="gameCanvas" width="300" height="600"></canvas>
<div class="info-panel">
<h2>スコア: <span id="score">0</span></h2>
<h3>次のブロック:</h3>
<canvas id="nextBlockCanvas" width="100" height="100"></canvas>
<button id="pauseButton">一時停止</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
※今回chat GPTが出してくれたHTMLコードです。
JavaScriptコード
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const nextCanvas = document.getElementById('nextBlockCanvas');
const nextCtx = nextCanvas.getContext('2d');
const scoreElement = document.getElementById('score');
const pauseButton = document.getElementById('pauseButton');
const ROWS = 20;
const COLS = 10;
const BLOCK_SIZE = 30;
let score = 0;
let isPaused = false;
let gameInterval;
// ブロックの形だけを定義
const shapes = [
[[1, 1, 1, 1]], // I型
[[1, 1], [1, 1]], // O型
[[0, 1, 1], [1, 1, 0]], // S型
[[1, 1, 0], [0, 1, 1]], // Z型
[[1, 1, 1], [0, 1, 0]], // T型
[[1, 1, 1], [1, 0, 0]], // L型
[[1, 1, 1], [0, 0, 1]] // J型
];
// ブロックに対応する色を定義
const colors = [
'cyan', // I型
'yellow', // O型
'green', // S型
'red', // Z型
'purple', // T型
'orange', // L型
'blue' // J型
];
// ピースを作成
function createPiece() {
const index = Math.floor(Math.random() * shapes.length);
return {
shape: shapes[index], // ランダムに形状を取得
color: colors[index], // ランダムに色を取得
x: Math.floor(COLS / 2) - Math.floor(shapes[index][0].length / 2),
y: 0
};
}
let board = createBoard();
let currentPiece = createPiece();
let nextPiece = createPiece();
// ゲームループ
function gameLoop() {
if (!isPaused) {
moveDown();
draw();
}
}
// ボードを作成
function createBoard() {
return Array.from({ length: ROWS }, () => Array(COLS).fill(0));
}
// ピースを描画
function drawPiece(piece, ctx, offsetX = 0, offsetY = 0) {
piece.shape.forEach((row, y) => {
row.forEach((value, x) => {
if (value) {
ctx.fillStyle = 'cyan';
ctx.fillRect((piece.x + x + offsetX) * BLOCK_SIZE, (piece.y + y + offsetY) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
ctx.strokeStyle = 'white';
ctx.strokeRect((piece.x + x + offsetX) * BLOCK_SIZE, (piece.y + y + offsetY) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
});
});
}
// ゲームを描画
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBoard();
drawPiece(currentPiece, ctx);
drawPiece(nextPiece, nextCtx, 0, 0);
}
// ボードを描画
function drawBoard() {
board.forEach((row, y) => {
row.forEach((value, x) => {
if (value) {
ctx.fillStyle = 'cyan';
ctx.fillRect(x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
ctx.strokeStyle = 'white';
ctx.strokeRect(x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
});
});
}
// ピースを下に移動
function moveDown() {
currentPiece.y++;
if (isCollision()) {
currentPiece.y--;
mergePiece();
currentPiece = nextPiece;
nextPiece = createPiece();
if (isGameOver()) {
clearInterval(gameInterval);
alert('Game Over');
}
}
}
// 衝突判定
function isCollision() {
return currentPiece.shape.some((row, y) =>
row.some((value, x) => {
if (value && (board[currentPiece.y + y] && board[currentPiece.y + y][currentPiece.x + x]) !== 0) {
return true;
}
return false;
})
);
}
// ピースをボードに統合
function mergePiece() {
currentPiece.shape.forEach((row, y) => {
row.forEach((value, x) => {
if (value) {
board[currentPiece.y + y][currentPiece.x + x] = value;
}
});
});
clearLines();
}
// ラインをクリア
function clearLines() {
let linesCleared = 0;
board = board.filter(row => {
if (row.every(value => value !== 0)) {
linesCleared++;
return false;
}
return true;
});
while (board.length < ROWS) {
board.unshift(Array(COLS).fill(0));
}
score += linesCleared * 100;
scoreElement.textContent = score;
}
// ゲームオーバー判定
function isGameOver() {
return board[0].some(value => value !== 0);
}
// 一時停止
pauseButton.addEventListener('click', () => {
isPaused = !isPaused;
pauseButton.textContent = isPaused ? '再開' : '一時停止';
});
// ゲーム開始
gameInterval = setInterval(gameLoop, 1000);
// キーボード操作を追加
document.addEventListener('keydown', (event) => {
if (isPaused) return; // 一時停止中は操作不可
switch (event.key) {
case 'ArrowLeft':
moveLeft();
break;
case 'ArrowRight':
moveRight();
break;
case 'ArrowDown':
moveDown();
break;
case 'ArrowUp':
rotatePiece();
break;
case ' ':
hardDrop();
break;
case 'p': // 一時停止
isPaused = !isPaused;
pauseButton.textContent = isPaused ? '再開' : '一時停止';
break;
}
draw(); // 動作後に再描画
});
// 左に移動
function moveLeft() {
currentPiece.x--;
if (isCollision()) {
currentPiece.x++;
}
}
// 右に移動
function moveRight() {
currentPiece.x++;
if (isCollision()) {
currentPiece.x--;
}
}
// 回転
function rotatePiece() {
const prevShape = currentPiece.shape;
currentPiece.shape = currentPiece.shape[0].map((_, index) =>
currentPiece.shape.map(row => row[index]).reverse()
);
if (isCollision()) {
currentPiece.shape = prevShape; // 衝突する場合は元に戻す
}
}
// ハードドロップ
function hardDrop() {
while (!isCollision()) {
currentPiece.y++;
}
currentPiece.y--; // 衝突前の位置に戻す
mergePiece();
currentPiece = nextPiece;
nextPiece = createPiece();
draw(); // ドロップ後の状態を描画
}
※今回chat GPTが出してくれたJavaScriptコードです。
cssコード
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.game-container {
display: flex;
}
canvas {
background-color: #333;
margin: 10px;
}
.info-panel {
display: flex;
flex-direction: column;
align-items: center;
}
button {
margin-top: 20px;
padding: 10px;
font-size: 16px;
}
※今回chat GPTが出してくれたcssコードです。
テストプレイ
コードを貼り付けた後は、上のRUNボタンでtestプレイしましょう。
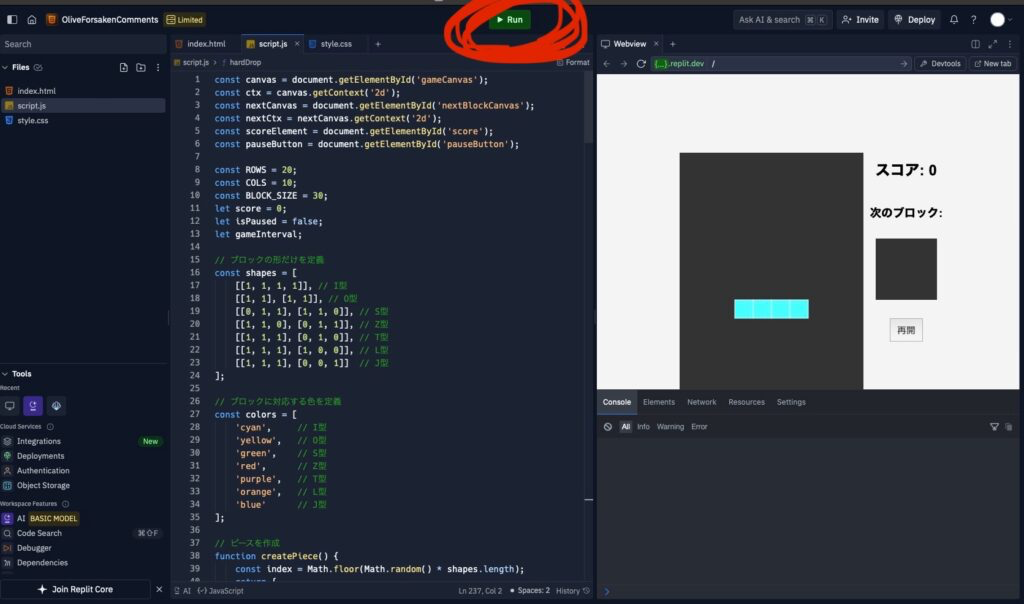
完成
初めだと、chatGPTが適切なコードを出してくれない場合もあります。
その場合の対処法は、間違っている部分をchatGPTに言いましょう。
※例
キーボードで操作できない場合、、、
命令文
キーボードで操作できない、操作できるようにコード編集して下さい。
それで、満足がいくゲームが出来たら完成です!!!
ゲームの公開先
Steam
-1-1024x521.png)
【 Steam 】は、国内関係なく国外でも有名で、有名なタイトルめちゃめちゃ入っているため一番お勧めします!!
その代わり、ゲームを公開するまでに前払い、厳しい審査などがある為簡単には公開はできません、、、
itch.io
-1024x523.png)
【 itch.io 】は、PWYW(Pay What You Want)と言うのを採用しており、ゲームの値段は作者が決めれます。
unityroom
-1024x523.png)
【 unityroom 】は、先ほどの項目で説明しましたが、Unityで作成したゲームをそのまま投稿できます。
App Store
-1024x525.png)
【 App Store 】IOS版のアプリといえばこれ!!
ですが、ゲームの投稿をする際。Apple Developer Programへの登録や審査などの難題なミッションをクリアしないとせっかく創り上げたゲームを投稿できません、、、
Google Play Store
-1024x524.png)
【 Google Play Store 】Androidはこれ!!
Google Play Storeはまだ審査などは簡単なんですけど、Google Play デベロッパー アカウントへの登録および支払い、アプリ登録などの手順を踏まないといけませんので、結構めんどくさいですね、、、
Freem!
-1024x523.png)
【 Freem! 】は、無料で遊べるPCゲームが結構ありますので、ゲーム公開する際は審査が通りやすいです。
PLiCy
-1-1024x524.png)
【 PLiCy 】は、PCとスマホでも遊べるゲームが多く公開されています。
※自動でスマホ版が公開されます。
コメント